Object-Oriented Programming
Java is an object-oriented programming language, meaning that it is based on the concept of "objects", which can contain code and data. For example, a red car would be an object with attributes, such as weight, color, position, and direction, and methods, which are functions such as drive and turn. A class is used to create objects, and essentially acts as a blueprint for objects.
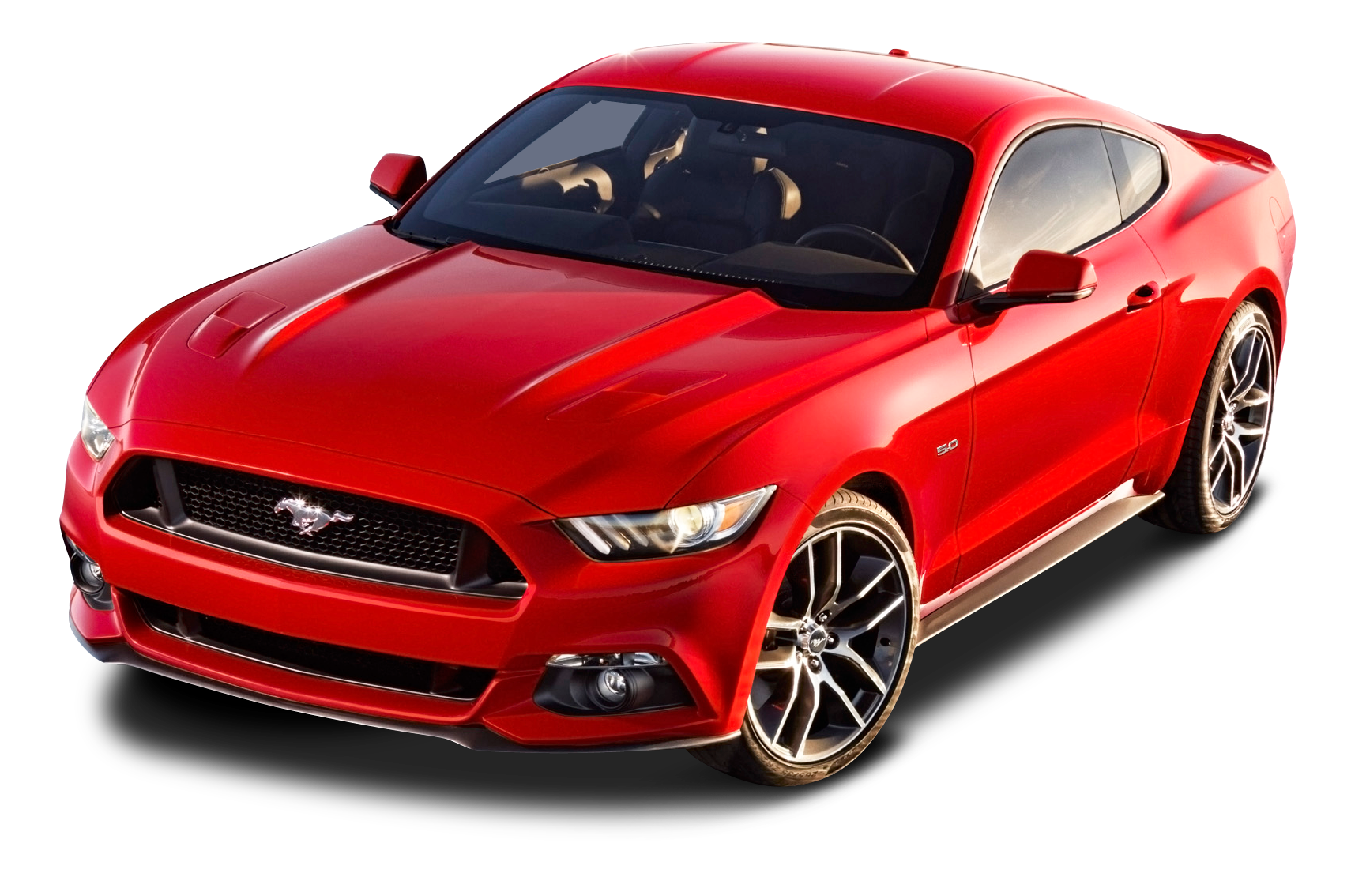
How are Classes Created?
Classes can be created with the class
keyword. The Car
class below recreates the previously mentioned car.
public class Car { // Car class
// Attributes
private int weight;
private String color;
private int position;
private char direction;
// Constructor - This is needed to make an object
public Car(int w, String c) { // Takes 2 inputs, w and c
weight = w; // Sets weight to w
color = c; // Sets color to c
position = 0;
direction = 'N';
}
// Methods
// Drive method
public void drive(int n) {
position += n;
}
// Turn methods
public void turnRight() {
switch (direction) {
case 'N': direction = 'E';
case 'E': direction = 'S';
case 'S': direction = 'W';
case 'W': direction = 'N';
}
}
public void turnLeft() {
switch (direction) {
case 'N': direction = 'W';
case 'E': direction = 'N';
case 'S': direction = 'E';
case 'W': direction = 'S';
}
}
}
How are Objects Created?
Once the class has been created, creating an object is trivial. Simply use the new
keyword to make an instance of the class
(an object), as such:
public static void main(String[] args) {
Car myCar = new Car(5, "red"); // Makes a new Car object called myCar with a weight of 5 and color "red"
}
Get and set methods can also be used to get and set the attributes of the object, for example:
public int getWeight() {
return weight;
}
public void setWeight(int w) {
weight = w;
}
public String getColor() {
return color;
}
public void setColor(String c) {
color = c;
}
Advantages of Object-Oriented Programming
Object-oriented programming allows for much more modularity and easier troubleshooting. It also allows for big projects to be broken down into smaller, more intuitive components, which can be very useful for applications such as software development and large scale operations such as cloud computing networks. Its modular nature also makes it a good choice when working in large groups, as it allows for roles to be divided clearly and easily.